- Teacher: varun barthwal
Hemvati Nandan Bahuguna Garhwal University
Available courses
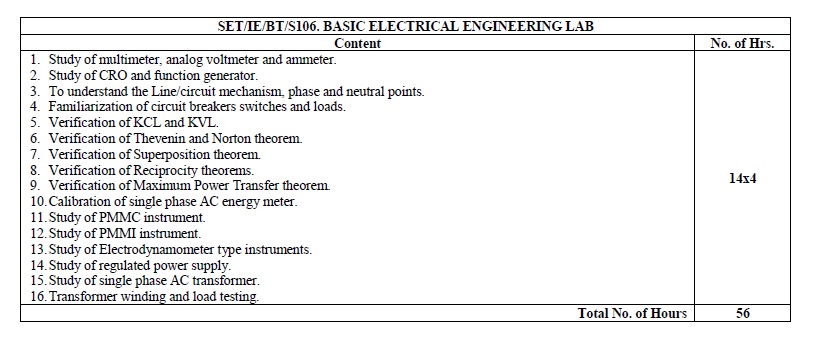
Course Objectives:
1. To impart basic knowledge of electrical quantities and provide working knowledge for the analysis of DC and AC circuits.
2. To provide the knowledge of various theorems like thevenin, norton, superposition etc.
- Teacher: Dr. Don Biswas Assistant Professor
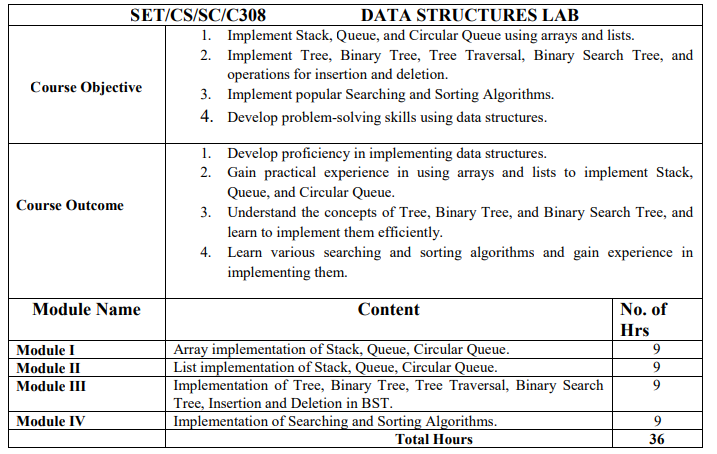
Course Objective
1. Implement Stack, Queue, and Circular Queue using arrays and lists.
2. Implement Tree, Binary Tree, Tree Traversal, Binary Search Tree, and operations for insertion and deletion.
3. Implement popular Searching and Sorting Algorithms.
4. Develop problem-solving skills using data structures.
Course Outcome
1. Develop proficiency in implementing data structures.
2. Gain practical experience in using arrays and lists to implement Stack, Queue, and Circular Queue.
3. Understand the concepts of Tree, Binary Tree, and Binary Search Tree, and learn to implement them efficiently.
4. Learn various searching and sorting algorithms and gain experience in
implementing them.
- Teacher: Prof. M.M.S. Rauthan Professor
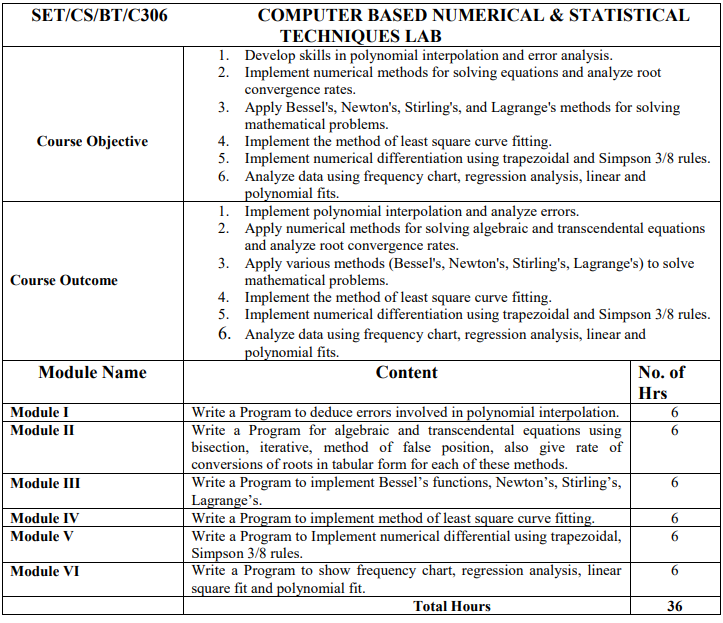
Course Objective
1. Develop skills in polynomial interpolation and error analysis.
2. Implement numerical methods for solving equations and analyze root convergence rates.
3. Apply Bessel's, Newton's, Stirling's, and Lagrange's methods for solving mathematical problems.
4. Implement the method of least square curve fitting.
5. Implement numerical differentiation using trapezoidal and Simpson 3/8 rules.
6. Analyze data using frequency chart, regression analysis, linear and polynomial fits.
Course Outcome
1. Implement polynomial interpolation and analyze errors.
2. Apply numerical methods for solving algebraic and transcendental equations and analyze root convergence rates.
3. Apply various methods (Bessel's, Newton's, Stirling's, Lagrange's) to solve mathematical problems.
4. Implement the method of least square curve fitting.
5. Implement numerical differentiation using trapezoidal and Simpson 3/8 rules.
6. Analyze data using frequency chart, regression analysis, linear and
polynomial fits.
- Teacher: Prof. M.M.S. Rauthan Professor
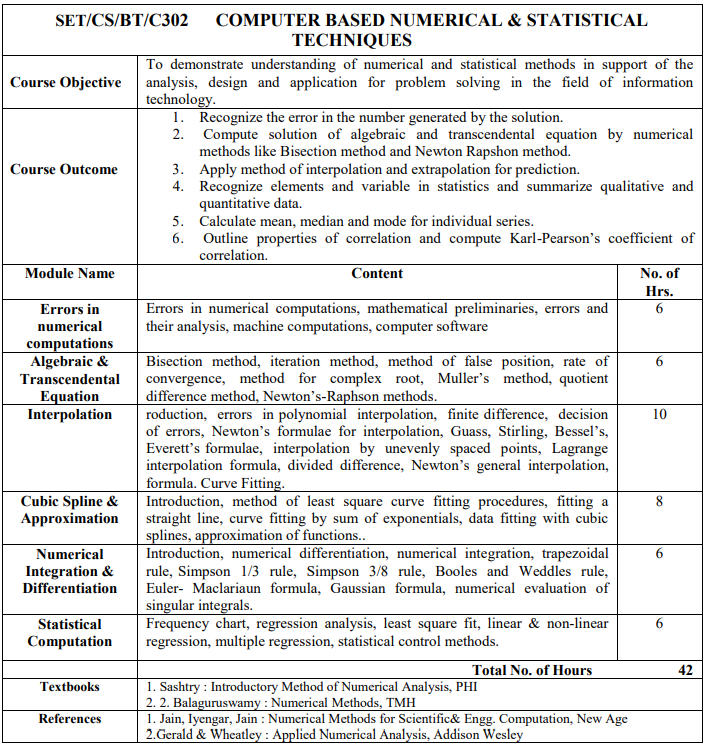
Course Objective : To demonstrate understanding of numerical and statistical methods in support of the analysis, design and application for problem solving in the field of information technology.
Course Outcome:
1. Recognize the error in the number generated by the solution.
2. Compute solution of algebraic and transcendental equation by numerical methods like Bisection method and Newton Rapshon method.
3. Apply method of interpolation and extrapolation for prediction.
4. Recognize elements and variable in statistics and summarize qualitative and quantitative data.
5. Calculate mean, median and mode for individual series.
6. Outline properties of correlation and compute Karl-Pearson‟s coefficient of
correlation.
- Teacher: Prof. M.M.S. Rauthan Professor
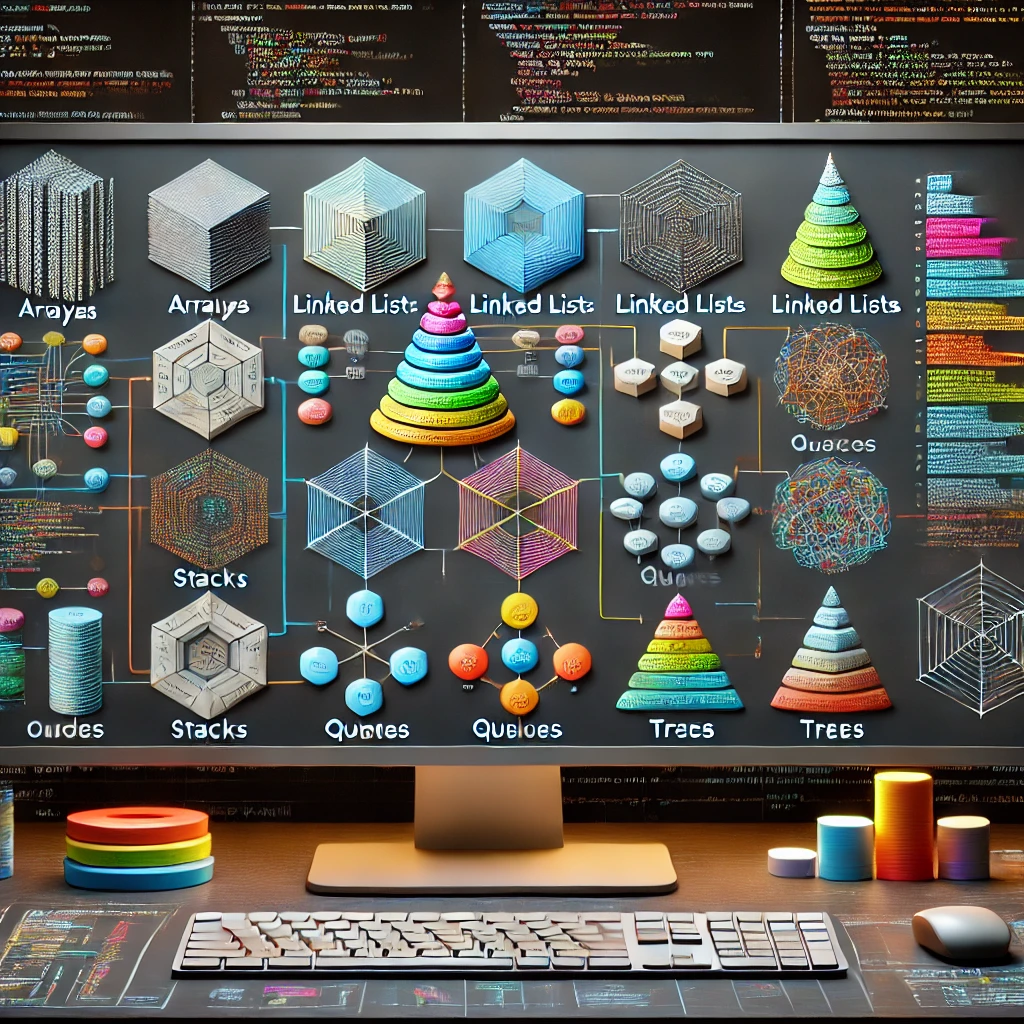
This course is designed to provide a comprehensive understanding of data structures, their implementations in C, and their applications in solving complex problems.
What You'll Learn:
- Introduction to Data Structures: Understand the basics of data organization and manipulation.
- Core Data Structures:
- Arrays: Sequential data storage and manipulation.
- Linked Lists: Dynamic memory management with singly, doubly, and circular linked lists.
- Stacks & Queues: Learn LIFO and FIFO concepts for data processing.
- Trees & Binary Trees: Organize hierarchical data efficiently.
- Graphs: Represent and navigate complex networks.
- Hash Tables: Fast data retrieval and storage using hashing techniques.
- Algorithm Integration: Implement sorting (bubble, merge, quick) and searching (binary, linear) algorithms.
- Practical Implementation: Write C code to create and optimize each data structure.
- Problem-Solving Skills: Apply data structures to real-world challenges like route planning, scheduling, and memory management.
- Teacher: Prof. M.M.S. Rauthan Professor
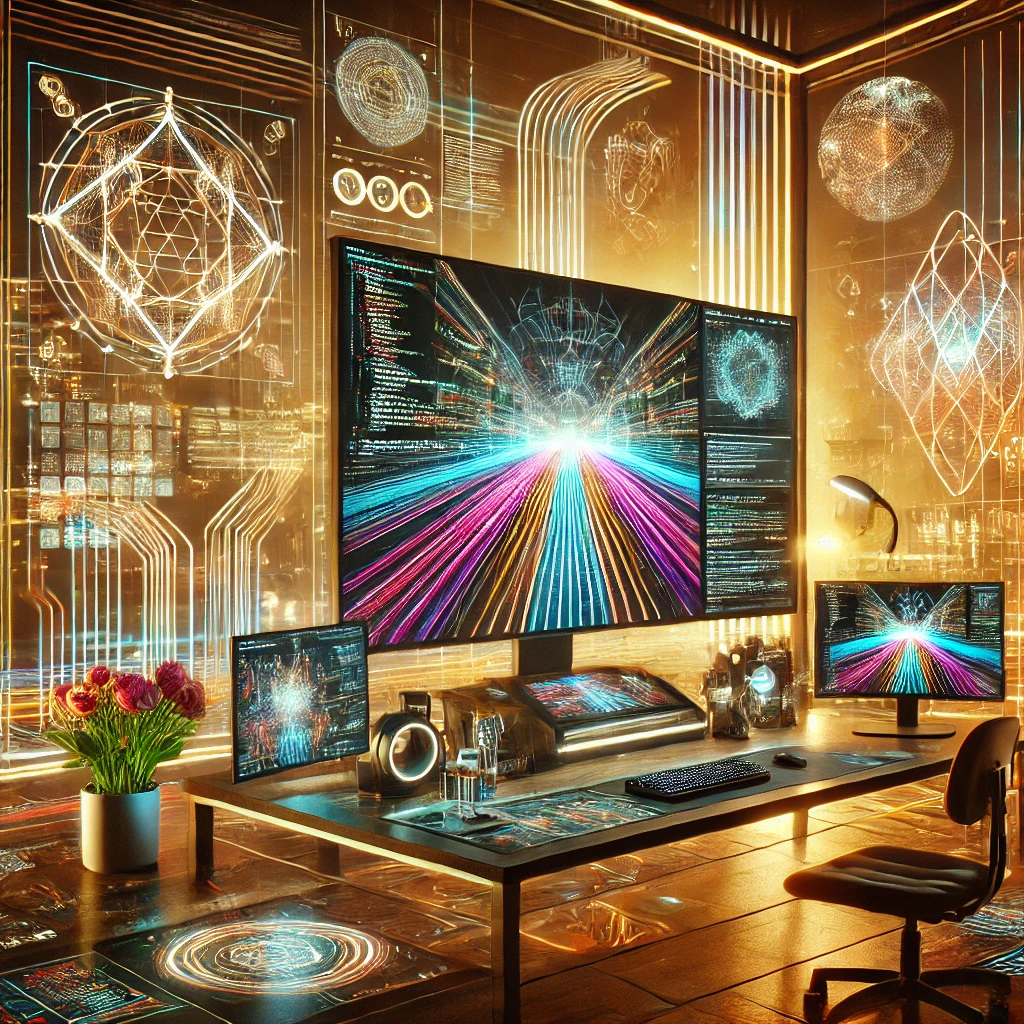
This course is designed for beginners and covers the foundational programming concepts, equipping you with the skills to write, debug, and execute code confidently.
What You'll Learn:
- Programming Basics: Understand variables, data types, and control structures.
- Problem-Solving Techniques: Break down complex problems into manageable solutions using algorithms.
- Coding Languages: Get hands-on experience with programming language C/ C++.
- Debugging and Optimization: Learn to identify and fix errors in your code effectively.
- Real-World Applications: Work on practical projects, such as creating games, automating tasks, and developing basic web applications.
Outcomes:
By the end of this course, you will be able to write functional programs, understand programming logic, and be prepared to advance to more specialized areas such as web development, data science, or software engineering.
- Teacher: varun barthwal
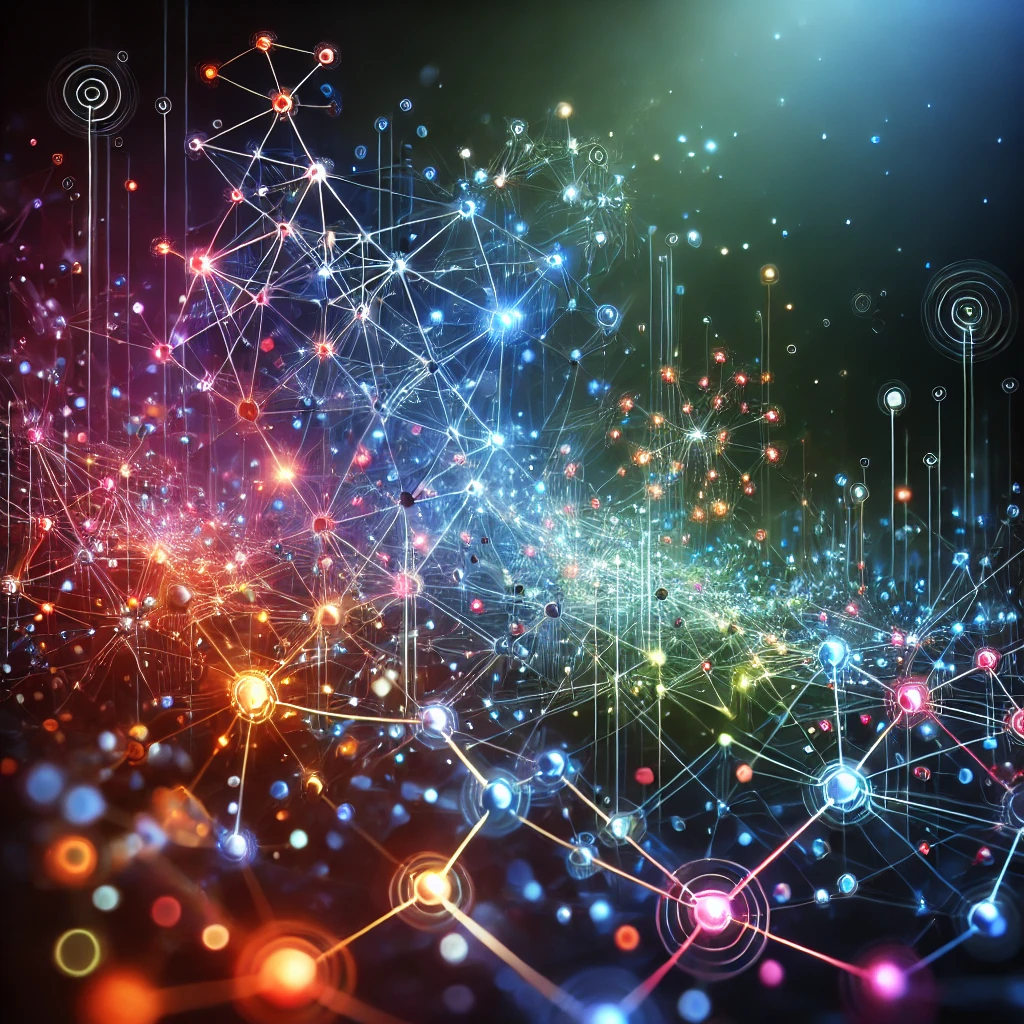
This course provides a comprehensive introduction to the concepts, techniques, and applications of graph theory.
What You'll Learn:
- Foundational Concepts: Understand the basics of graphs, including vertices, edges, paths, cycles, and connectivity.
- Types of Graphs: Learn about directed graphs, undirected graphs, weighted graphs, bipartite graphs, and more.
- Graph Algorithms: Explore key algorithms like shortest path (Dijkstra's and Bellman-Ford), minimum spanning tree (Kruskal's and Prim's), and depth-first and breadth-first searches.
- Applications of Graph Theory: Discover real-world uses in areas like computer networks.
By the end of this course, you’ll be able to analyze and solve problems using graph theory concepts, implement graph algorithms, and apply graph-based solutions in diverse fields such as data science, AI, and operations research.
- Teacher: varun barthwal
this is test course
- Teacher: varun barthwal
- Teacher: varun barthwal
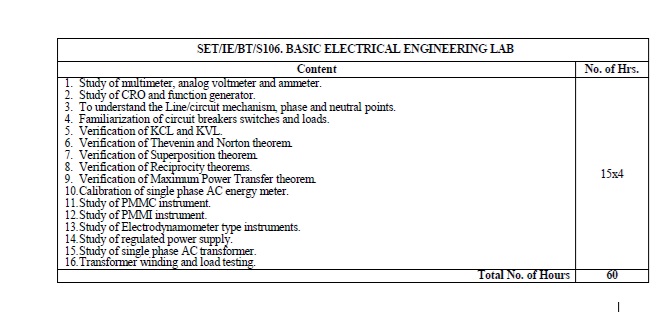
Course Objectives:
1. To impart basic knowledge of electrical quantities and provide working knowledge for the analysis of DC and AC circuits.
2. To provide the knowledge of various theorems like thevenin, norton, superposition etc.
- Teacher: Dr. Don Biswas Assistant Professor
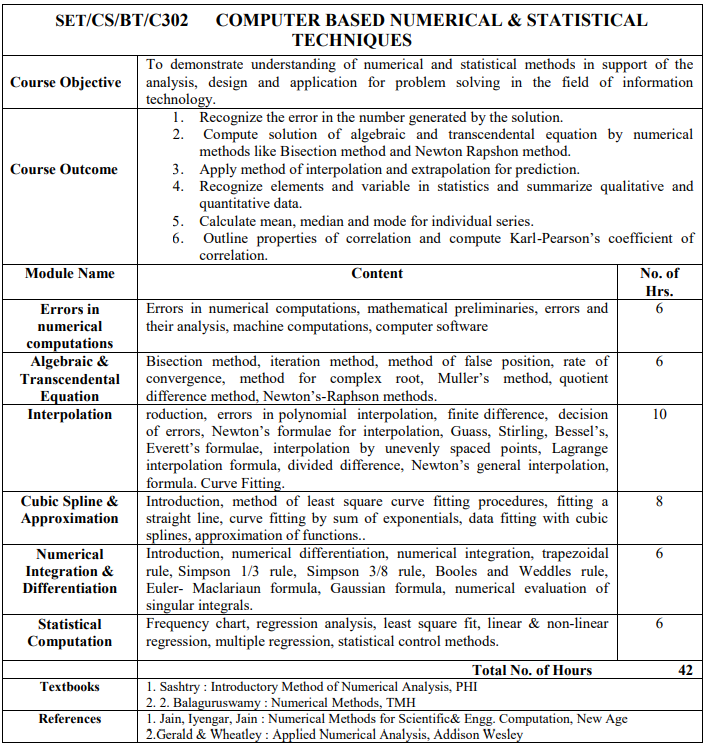
Course Objective: To demonstrate understanding of numerical and statistical methods in support of the analysis, design and application for problem solving in the field of information technology.
Course Outcome :
1. Recognize the error in the number generated by the solution.
2. Compute solution of algebraic and transcendental equation by numerical methods like Bisection method and Newton Rapshon method.
3. Apply method of interpolation and extrapolation for prediction.
4. Recognize elements and variable in statistics and summarize qualitative and quantitative data.
5. Calculate mean, median and mode for individual series.
6. Outline properties of correlation and compute Karl-Pearson‟s coefficient of
correlation.
- Teacher: Prof. M.M.S. Rauthan Professor
Write a Shell script that accepts a filename, starting and ending line numbers as arguments and displays all the lines between the given line numbers.
Write a Shell script to show functioning of for loop in shell programming.
Write a shell script using while loop.
Write a shell script using if else statement
Write a Shell script to list all the files in a directory
Write a Shell script to find whether a number is even or odd
Write a Shell script to find the factorial of a given integer
Write a shell script that computes the gross salary of an employee according to the following rules: (The basic salary is entered interactively through the keyboard.) I. If basic salary is < 1500 then HRA-10% of the basic and DA =90% of the basic. II. If the basic salary is >=1500 then HRA-Rs500 and DA-98% of the basic
Write a C program to create a zombie process.
Write a shell script which receives two file names as arguments. It should check whether the two file contents are the same or not. If they are the same then the second file should be deleted.
Describe some Internal commands
Describe some External commands
* Introduction of Full Stack Development using Spring boot
* Using bootstrapping in Spring boot
* Working with Tomcat deployment
(Besides these additional experiments can be included to give hands on experience to students. Students
can be provided opportunity to work on any Information System to give them better understanding of
Information System)
Course Outcome:
Through completion of the Certificate course in Information Technology program, students will:
1. Develop information technology solutions by evaluating user requirements in the systems development environment.
2. Apply knowledge of IT requirements for technology solutions in cutting edges applications.
3. Analyze a problem and identify and define the computing requirements for the appropriate solutions.
4. Create, select and apply appropriate techniques, resources, and modern engineering and IT tools.
1. Creating ER diagrams and Schema Diagrams of real world problems.
2. Creating tables and data population.
3. Writing SQL queries using following operators: (a) Logical operators (=,<,>,etc.). (b) SQL operators (Between…. AND, IN(List), Like, ISNULL and also with negating expressions ). (c) Set Operators(UNION, INTERSECT, and MINUS, etc.).
4. Writing SQL queries using Character, Number, Date and Group functions.
5. Writing SQL queries for extracting data from more than one table (Equi-Join, Non-EquiJoin , Outer Join)
6. Creating VIEWS using SQL and performing operation on it.
7. Writing ASSERTIONS using SQL
8. Writing programs using PL/SQL.
9. Use Concepts for ROLL BACK, COMMIT & CHECK POINTS.
10. Write queries using CURSORS.
11. Write TRIGGRS using PL/SQL
12. Create FORMS and REPORTS.
1. Divide and conquer method (quick sort, merge sort, Strassen’s matrix multiplication)
2. Greedy method (knapsack problem, job sequencing, optimal merge patterns, minimal spanning trees).
3. Dynamic programming (multistage graphs, OBST, 0/1 knapsack, traveling salesperson problem).
4. Back tracking (n-queens problem, graph coloring problem, Hamiltonian cycles).
5. Sorting : Insertion sort, Heap sort, Bubble sort
6. Searching : Sequential and Binary Search
7. Selection : Minimum/ Maximum, Kth smallest element
* Using bootstrapping in Spring boot, Working with Tomcat deployment
* Spring Boot- code structure ,logging ,Building restful web services
* (Besides these additional experiments can be included to give hands on experience to students. Students can be provided opportunity to work on any Information System to give them better understanding of Information System)
Working with Microsoft office (word, excel, power point, access)
Use of Search Engine and World Wide Web, Creation of email id and working with email, Use of FTP service
Basics of Cloud computing, Internet of things (IoT) and Data Science
(Besides these additional experiments can be included to give hands on experience to students. Students can be provided opportunity to work on any Information System to give them better understanding of Information System)
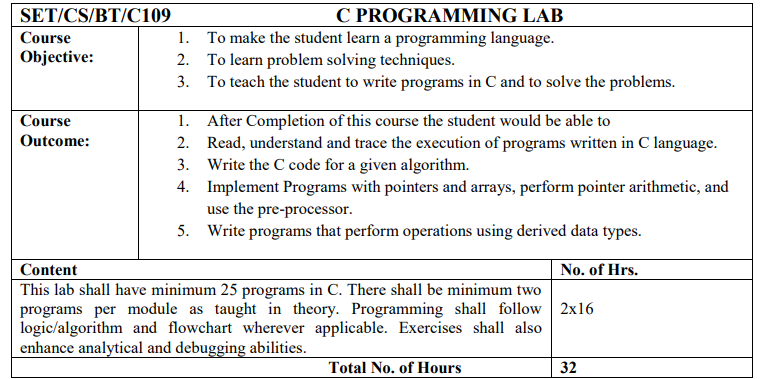
This lab shall have minimum 25 programs in C. There shall be minimum two
programs per module as taught in theory. Programming shall follow
logic/algorithm and flowchart wherever applicable. Exercises shall also
enhance analytical and debugging abilities.
3. Biopolymers and their Molecular Weights
Evaluation of size, shape, molecular weight and extent of hydration of biopolymers by various experimental techniques. Sedimentation equilibrium, hydrodynamic methods, diffusion, sedimentation velocity, viscosity, electrophoresis and rotational motions.
- Teacher: Dr. Pawan Kumar Assistant Professor
Unit I
Basics
Importance of polymers. Basic concepts: Monomers, repeat units, degree of polymerization. Linear, branched and network polymers. Classification of polymers. Polymerization: condensation, addition, radical chain, ionic and co-ordination and co-polymerization. Polymerization conditions and polymer reactions. Polymerization n homogenous and heterogeneous systems.
Unit II
Polymer Characterization
Polydispersion-average molecular weight concept. Number, weight and viscosity average molecular weights. Polydispersity and molecular weight distribution. The practical significance of molecular weight. Measurement of molecular weights. End-group, viscosity, light scattering, osmotic and ultracentrifugation methods. Analysis and testing of polymers-chemical analysis of polymers, spectroscopic methods, X-ray diffraction study. Microscopy. Thermal analysis and physical testing testing strength. Fatigue, impact. Tear resistance. Hardness and abrasion resistance.
- Teacher: Dr. Pawan Kumar Assistant Professor
Unit I
Theoretical and Computational Treatment of Atoms and Molecules,
Hartree-Fock Theory Review of the principles of quantum mechanics, Born-Oppenheimer approximation. Slater-Condon rules, Hartree-Fock equation, Koopmans and Brillouin theories, Roothan equation, Gaussian basis sets.
- Teacher: Dr. Pawan Kumar Assistant Professor
Electrochemistry
Electrochemistry of solutions, Debye-Huckel, Onsager treatment and its extension, ion solvent interactions.Thermodynamics of electrified interface equations. Structure of electrified interfaces. Guoy Chapman, Stern. Over potentials, exchange current density, derivation of Butler-Volmer equation, Tafel plot.
Semiconductor interfaces-theory of double layer at semiconductor, electrolyte solution interfaces, structure of double layer interfaces. Electrocatalyis – influence of various parameters. Hydrogen electrode. Bioelctrochemistry, threshold membrane phenomena. Polarography theory, Ilkovic equation, half wave potential and its significance. Introduction to corrosion, homogeneous theory, forms of corrosion, corrosion monitoring and prevention methods.
Unit I Electron Spin Resonance Spectroscopy
Principle and theory, Kramer degeneracy, g factor, electron-nuclear coupling (hyperfine structure), line shape and width, Mc Connell relationship, endor and eldor, electron-electron coupling. Techniques of measurement, application of ESR to organic free radicals and to transitional metal complexes (having and unpaired electron) including biological systems.
Unit II Nuclear Magnetic Resonance Spectroscopy
(a). Chemical shift values for protons bonded to carbon (aliphatic, olelinic, aldehydic and aromatic)
and other nuclei (alcohols, phenols, carboxylic acids, amines, amides), chemical exchange, effects
of deuteration, Karplus curve-variation of coupling constant with dihedral angle.
(b). Carbon-13 NMR Spectroscopy
General consideration, chemical shift (aliphatic, olefinic, alkyne, aromatic, heteroaromatic and
carbonyl compound), coupling constants.
(c). Nuclear Quadrupole Resonance: Principle, Theory and applications
- Teacher: Dr. Rohit Mahar Assistant Professor
- Teacher: Dr. Bibhisan Roy Assistant Professor
Unit III Magnetic Resonance Spectroscopy
Nuclear Magnetic Resonance Spectroscopy
Nuclear spin, nuclear resonance, saturation, shielding of magnetic nuclei, chemical shift and its
measurement, factor influencing chemical shift, deshielding, spin-spin interaction, factors influencing
coupling constant ‘J’. Classification (ABX, AMX, ABC, A2B2 etc.), spin decoupling, basic ideas about
instrument, NMR studies of nuclei other than proton13C, 19F and 31P. FT NMR, advantages of FT NMR,
use of NMR in medical diagnostics.
- Teacher: Dr. Rohit Mahar Assistant Professor
Unit-III
Non-Equilibrium Thermodynamics
Non-Equilibrium Thermodynamics
Thermodynamic criteria for non-equilibrium states, entropy production and entropy flow, entropy
balance equations for different irreversible processes (e.g., heat flow, chemical reaction etc.)
transformations of the generalized fluxes and forces, non-equilibrium stationary states,
phenomenological equations, microscopic reversibility and Onsager’s reciprocity relations,
electrokinetic phenomena, diffusion, electric conduction, irreversible thermodynamics for biological
systems, coupled reactions.
- Teacher: Dr. Pawan Kumar Assistant Professor
Unit IV
Chromatographic Methods
Principle, instrumentation and applications of gas liquid chromatography and HPLC. Ion exchange chromatography: cationic and anionic exchanges and their applications. Van-Deemter equation (no derivation), concept about HEPT-plate theory and rate theory. Applications.
Unit V
Radio Analytical Methods
Basic principles and types of measuring instruments, isotope dilution techniques: principle of operations and uses. Applications.
- Teacher: Dr. Shikha Dubey Assistant Professor
Unit II
Statistical Thermodynamics
Concept of distribution, thermodynamic probability and most probable distribution. Ensemble
averaging, postulates of ensemble averaging. Canonical, grand canonical and microcanonical ensembles,
corresponding distribution laws- (using Lagrange’s method of undetermined multipliers).
Partition functions- translational, rotational, vibrational and electronic partition functions.
Calculation of thermodynamic properties in terms of partition functions. Applications of partition
functions.
Unit I
Electronic Spectra & Magnetic Properties of Transition Metal Complexes.
Unit III
Metal Clusters
Unit IV
Silicates
- Teacher: Dr. Bhaskaran Assistant Professor
Organic
Chemistry - I
Unit I
Nature of Bonding in Organic Molecules
Unit II
Stereochemistry
Unit III
Reaction Mechanism : Structure and Reactivity
Unit IV
Aliphatic Nucleophilic Substitution
Unit V
Aliphatic Electrophilic Substitution
Total Unit - 5
Unit I - Disconnection Approach
Unit II - Protecting Groups
Unit III - One Group and Two Group C-C Disconnections
Unit IV - Determination of Reaction Mechanism
Unit V - Photochemical Reactions
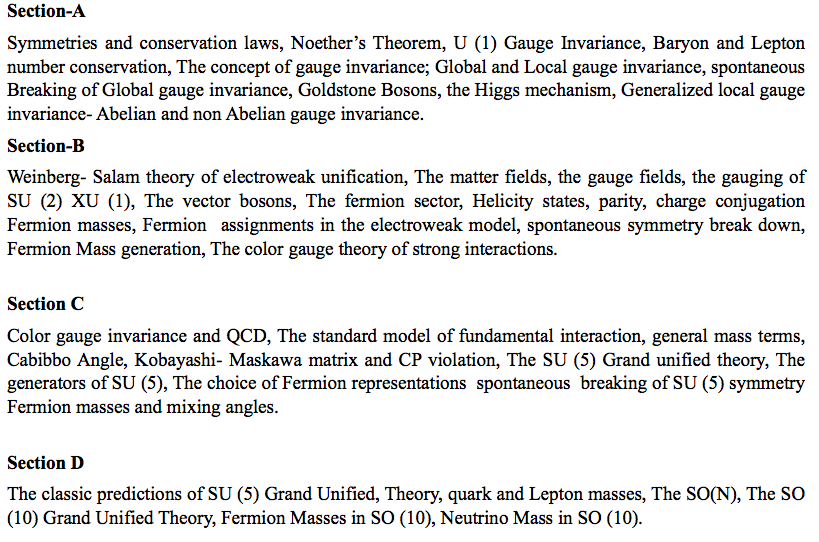
This course is designed to teach the fundamental particles and forces of nature. The first section of the course includes Noether's theorem, associated symmetries and conservation laws, Guage invariance, and Higgs mechanism. The second section teaches the theory of electroweak unification, SU(2)xU(1) gauging, Fermions in electroweak theory, their mass generation, and color gauge theory of strong interactions. The last two sections delve deep into the QCD, Standard Model of particle physics, SU(5) Grand unified theory, and SO(10) theory.
- Teacher: Dr. Vivek Sharma Assistant Professor
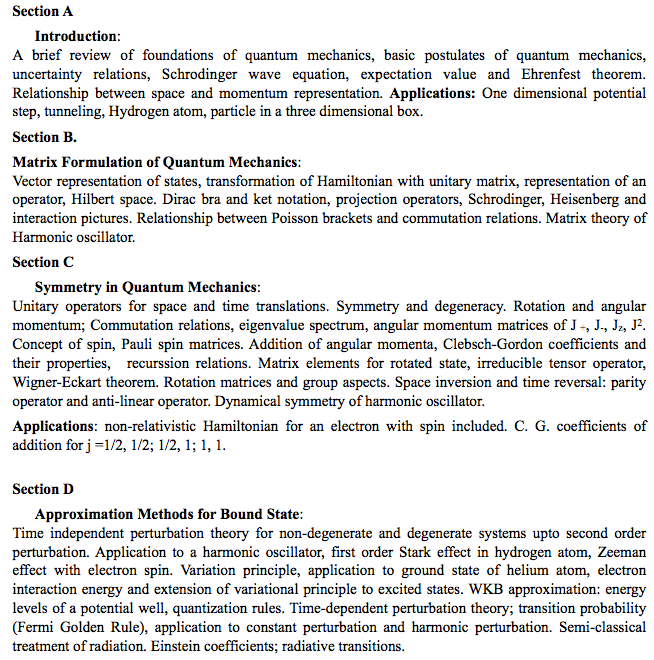
- Teacher : Dr. Vivek Sharma Assistant Professor
UNIT-I 07 Hours
Preformulation Studies: Introduction to preformulation, goals and objectives, study of
physicochemical characteristics of drug substances.
a. Physical properties: Physical form (crystal & amorphous), particle size, shape, flow
properties, solubility profile (pKa, pH, partition coefficient), polymorphism
b. Chemical Properties: Hydrolysis, oxidation, reduction, racemisation, polymerization
BCS classification of drugs & its significant
Application of preformulation considerations in the development of solid, liquid oral and
parenteral dosage forms and its impact on stability of dosage forms.
UNIT-II 10 Hours
Tablets:
a. Introduction, ideal characteristics of tablets, classification of tablets. Excipients,
Formulation of tablets, granulation methods, compression and processing problems.
Equipments and tablet tooling.
b. Tablet coating: Types of coating, coating materials, formulation of coating
composition, methods of coating, equipment employed and defects in coating.
c. Quality control tests: In process and finished product tests
Liquid orals: Formulation and manufacturing consideration of syrups and elixirs
suspensions and emulsions; Filling and packaging; evaluation of liquid orals
official in pharmacopoeia
UNIT-III 08 Hours
Capsules:
a. Hard gelatin capsules: Introduction, Production of hard gelatin capsule shells. size
of capsules, Filling, finishing and special techniques of formulation of hard gelatin
capsules, manufacturing defects. In process and final product quality control tests
for capsules.
b. Soft gelatin capsules: Nature of shell and capsule content, size of
capsules,importance of base adsorption and minim/gram factors, production, in
process and final product quality control tests. Packing, storage and stability testing
of soft gelatin capsules and their applications.
Pellets: Introduction, formulation requirements, pelletization process, equipments for
manufacture of pellets
UNIT-IV 10 Hours
Parenteral Products:
a. Definition, types, advantages and limitations. Preformulation factors and essential
requirements, vehicles, additives, importance of isotonicity
b. Production procedure, production facilities and controls,
aseptic processing
c. Formulation of injections, sterile powders, large volume parenterals and
lyophilized products.
d. Containers and closures selection, filling and sealing of ampoules, vials and infusion
fluids. Quality control tests of parenteral products.
Ophthalmic Preparations: Introduction, formulation considerations; formulation of eye
drops, eye ointments and eye lotions; methods of preparation; labeling, containers;
evaluation of ophthalmic preparations
UNIT-V 10 Hours
Cosmetics: Formulation and preparation of the following cosmetic preparations:
lipsticks, shampoos, cold cream and vanishing cream, tooth pastes, hair dyes and
sunscreens.
Pharmaceutical Aerosols: Definition, propellants, containers, valves, types of aerosol
systems; formulation and manufacture of aerosols; Evaluation of aerosols; Quality
control and stability studies.
Packaging Materials Science: Materials used for packaging of pharmaceutical products,
factors influencing choice of containers, legal and official requirements for containers,
stability aspects of packaging materials, quality control tests.
- Teacher: Dr. Mona Semalty Assistant Professor
Scope: This subject is designed to impart fundamental knowledge on the structure, chemistry, and therapeutic value of drugs. The subject emphasizes the structure-activity relationships of drugs, the importance of physicochemical properties, and the metabolism of drugs. The syllabus also emphasizes on chemical synthesis of important drugs under each class.
Objectives: Upon completion of the course, the student shall be able to
- Understand the chemistry of drugs with respect to their pharmacological activity.
- Understand the drug metabolic pathways, adverse effects, and therapeutic value of drugs.
- Know the Structural Activity Relationship (SAR) of different classes of drugs.
- Write the chemical synthesis of some drugs.
BP809ET. COSMETIC SCIENCE(Theory); 45Hours
UNIT I 10Hours
Classification of cosmetic and cosmeceutical products
Definition of cosmetics as per Indian and EU regulations, Evolution of cosmeceuticals
from cosmetics, cosmetics as quasi and OTC drugs
Cosmetic excipients: Surfactants, rheologymodifiers, humectants, emollients,
preservatives. Classification and application
Skin: Basic structure and function of skin.
Hair: Basic structure of hair. Hair growth cycle.
Oral Cavity: Common problem associated with teeth and gums.
UNIT II 10 Hours
Principles of formulation and building blocks of skin care products:
Face wash,
Moisturizing cream, Cold Cream, Vanishing cream and their advantages and
disadvantages.Application of these products in formulation of cosmecuticals.
Antiperspants & deodorants- Actives & mechanism of action.
Principles of formulation and building blocks of Hair care products:
Conditioning shampoo, Hair conditioner,anti-dandruff shampoo.
Hair oils.
Chemistry and formulation of Para-phylene diamine based hair dye.
Principles of formulation and building blocks of oral care products:
Toothpaste for bleeding gums, sensitive teeth. Teeth whitening, Mouthwash.
UNIT III 10 Hours
Sun protection, Classification of Sunscreens and SPF.
Role of herbs in cosmetics:
Skin Care: Aloe and turmeric
Hair care: Henna and amla.
Oral care: Neem and clove
Analytical cosmetics: BIS specification and analytical methods for shampoo, skincream and toothpaste.
UNIT IV 08 Hours.
Principles of Cosmetic Evaluation: Principles of sebumeter, corneometer. Measurement of TEWL, Skin Color, Hair tensile strength, Hair combing properties Soaps,and syndet bars. Evolution and skin benfits.
UNIT V 07 Hours
Oily and dry skin, causes leading to dry skin, skin moisturisation. Basic understanding of
the terms Comedogenic, dermatitis.
Cosmetic problems associated with Hair and scalp: Dandruff, Hair fall causes
Cosmetic problems associated with skin: blemishes, wrinkles, acne, prickly heat and
body odor.
Antiperspirants and Deodorants- Actives and mechanism of action
References
1) Harry’s Cosmeticology, Wilkinson, Moore, Seventh Edition, George Godwin.
2) Cosmetics – Formulations, Manufacturing and Quality Control, P.P. Sharma, 4th
Edition, Vandana Publications Pvt. Ltd., Delhi.
3) Text book of cosmelicology by Sanju Nanda & Roop K. Khar, Tata Publishers.
- Teacher: Dr. Mona Semalty Assistant Professor
BP 704T: NOVEL DRUG DELIVERY SYSTEMS (Theory)
45 Hours
Scope: This subject is designed to impart basic knowledge on the area of novel drug delivery systems.
Objectives:
Upon completion of the course, student shall be able
1. To understand various approaches for the development of novel drug delivery systems.
2. To understand the criteria for selection of drugs and polymers for the development of Novel drug delivery systems, their formulation and evaluation.
- Teacher: Dr. Ajay Semalty Assistant Professor
Applied Anthropology for the Students of MA/MSc III Semester
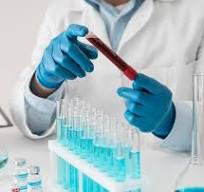
1. Disorders of Carbohydrate Metabolism - Diabetes mellitus, glucose and galactose tolerance tests, sugar levels in blood, renal threshold for glucose, factors influencing blood glucose level, glycogen storage diseases, pentosuria, galactosemia.
2. Disorders of Lipids – Plasma lipoproteins, cholesterol, triglycerides and phospholipids in health and disease, hyperlipidemia, hyperlipoproteinemia, Gaucher’s disease, Tay-Sach’s and Niemann-Pick disease, ketone bodies, Abetalipoproteinemia.
3. Inborn Errors of metabolism – Phenylketonuria, alkaptonuria, albinism, tyrosinosis, maple syrup urine disease, Lesch-Nyhan syndrome, sickle cell anemia, histidinemia.
4. Disorders of liver and kidney – Jaundice, fatty liver, normal and abnormal functions of liver and kidney. Inulin and urea clearance.
5. Electrolytes and acid-base balance – Regulation of electrolyte content of body fluids and maintenance of pH, reabsorption of electrolytes.
6. Diagnostic Enzymes – Enzymes in health and diseases. Biochemical diagnosis of diseases by enzyme assays – SGOT, SGPT, CPK, cholinesterase, LDH.
7. Blood Clotting – Disturbances in blood clotting mechanism – hemorrhagic disorders – hemophilia, von Willebrand’s disease, purpura, Rendu-Osler-Werber disease, thrombotic thrombocytopenic purpura, disseminated intravascular coagulation, acquired prothrombin complex disorders, circulating anticoagulants.
Cancer – Cellular differentiation, carcinogens and cancer therapy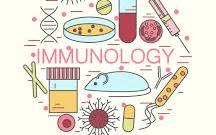
1. Introduction to immune system – Innate and acquired immunity. Structure and functions of primary and secondary lymphoid organs.
2. Cells involved in immune responses – Lymphoid cells (B-lymphocytes, T-lymphocytes and Null cells), mononuclear cells (phagocytic cells and their killing mechanisms), granulocytic cells (neutrophils, eosinophils and basophils), mast cells and dendritic cell.
3. Nature of antigen and antibody – Immunogenicity vs antigenicity, factors influencing immunogenicity, epitopes, haptens, adjuvants and mitogens. Classification, fine structure and functions of immunoglobulins, antigenic determinants on immunoglobulins, isotypic, allotypic and ideotypic variants.
4. Generation of Diversity in Immune system – Clonal selection theory - concept of antigen specific receptor. Organization of immunoglobulin genes: generation of antibody diversity, T-cell receptor diversity.
5. Immune effector Mechanisms – Kinetics of primary and secondary immune responses, complement activation and its biological consequences, cytokines and co-stimulatory molecules: role in immune responses, Antigen processing and presentation. Cell signaling – Role of MAP kinases.
6. Major histocompatibility complex (MHC) genes and products – Polymorphism of MHC genes, role of MHC antigens in immune responses, MHC antigens in transplantation.
7. Measurement of antigen–antibody interactions – Agglutination, precipitation and opsonization, gel diffusion (Ouchterlony double immunodiffusion and Mancini’s Radial immunodiffusion), immunoblotting, RIA, ELISA and ELISPOT.
8. Tolerance vs activation of immune system – Immune tolerance, hypersensitivity (Types I, II, III, IV).
Disorders of immune system – Autoimmunity, congenital immunodeficiencies, acquired immunodeficiencies.UNIT 2: FUNGI
- Teacher: Dr. Anant Kumar Assistant Professor
Semester III (July to November)
SLS/HAB/E02C Fishery Science (Theory)
SLS/HAB/E03 Lab course II
Semester I (July to November)
SLS/HAB/C002 Taxonomy & Systematics (Theory)
SLS/HAB/C005 Lab course I
- Teacher: Dr. Koshal Kumar Assistant Professor
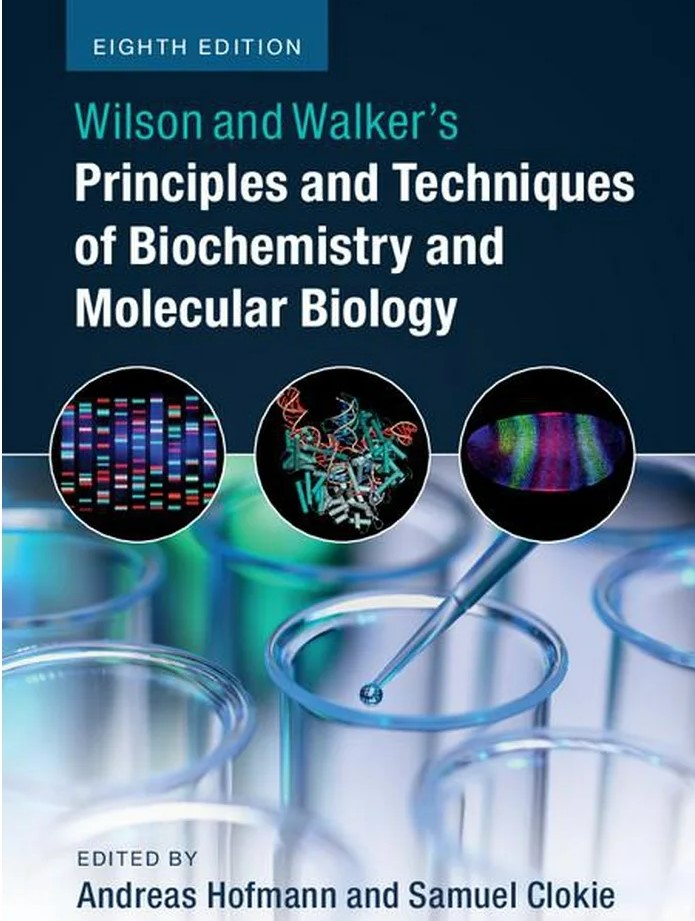
SLS/MIC/C009: BIOLOGICAL TECHNIQUES (M.Sc. Even Semester)
Unit I: Microscopy and Biosensors Microscopy (Principles and applications): Light, phase contrast, fluorescence and confocal microscopy, Scanning and transmission electron microscopy; Biosensors: Introduction and principles, First, second and third generation instruments, Cell based biosensors, Enzyme immunosensors, DNA biosensor.
Unit II: Centrifugation Basic principle and applications of centrifugation; Centrifugal force; Sedimentation rate; Sedimentation coefficient; Common centrifuges used in laboratory (Clinical, micro, high speed, ultra and industrial centrifuges); Types of rotors (Fixed- angle, swinging bucket and continuous tubular); Types of centrifugation (Principle and applications): Preparative (Differential and density gradient centrifugation) and analytical centrifugation.
Unit III: Chromatography General principle and applications of chromatography; Types of chromatography (Principles and applications): Adsorption chromatography, Ion exchange chromatography, Affinity chromatography, Size exclusion chromatography, Thin layer chromatography, Gas chromatography, High pressure liquid chromatography (HPLC), Supercritical fluid chromatography.
Unit IV: Electrophoretic Techniques General principle and applications of electrophoresis; Types of electrophoresis (Principles and applications): Paper electrophoresis, Moving boundary electrophoresis, Isotachophoresis, Agarose gel electrophoresis, Polyacrylamide gel electrophoresis (SDS-PAGE, Native-PAGE, Denaturing-PAGE and Reducing-PAGE), Isoelectric focusing (IEF), Pulse field gel electrophoresis (PFGE), Disc gel electrophoresis.
Unit V: Spectroscopy and Radiotracer Techniques
Spectroscopic methods (Principle and applications): UV, Visible, IR, NMR, Fluorescence, ESR,
Atomic absorption, CD, ORD and Raman Spectroscopy; Mass Spectrometry: Principles and
application of MALDI-MS, Ionization methods; Radiotracer techniques: Applications of
radioisotopes in biology, Properties and units of radioactivity, Radioactive isotopes and half-life,
Safety rules in handling of radioisotopes, Measurement of radioactivity (GM counter, gamma
counter, wilson cloud chamber and liquid scintillation counter), Autoradiography: Principle and
its applications.
- Teacher: Dr. Digar Singh Assistant Professor
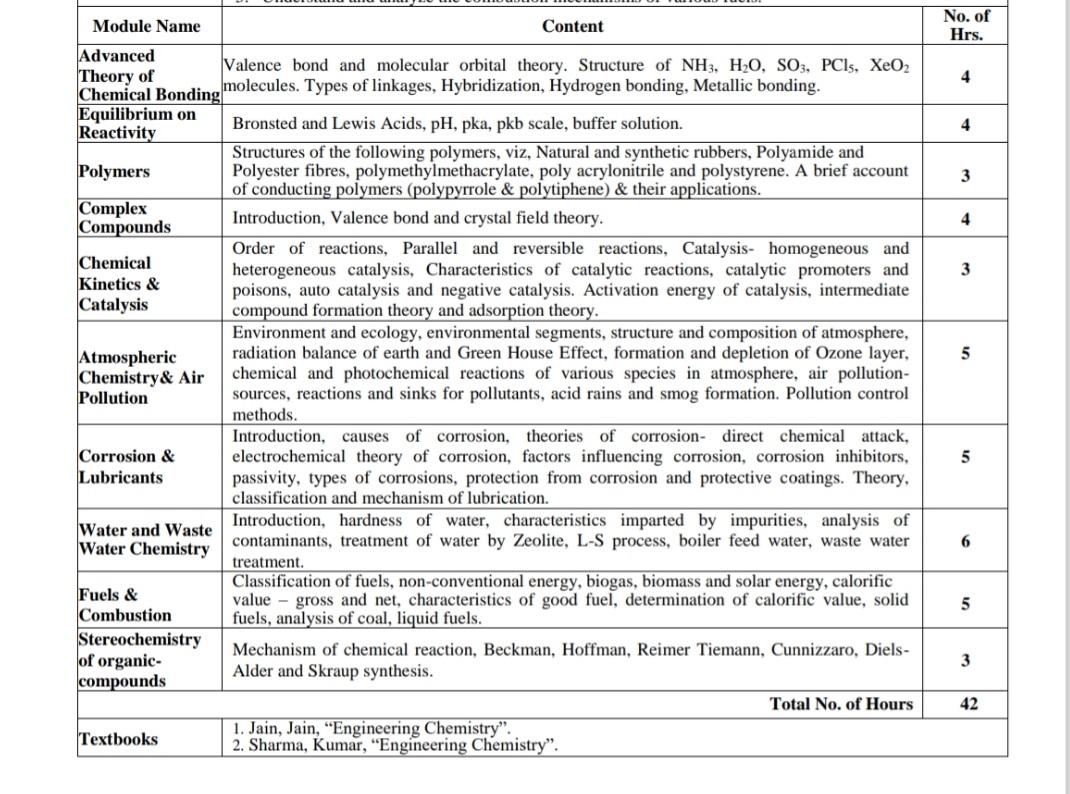
Course Objective
1. Apply the electrochemical principles in batteries, understand the fundamentals of corrosion.
2. Analysis of water for its various parameters and its significance in industrial and domestic Applications.
3. Analyze microscopic chemistry in terms of atomic, molecular orbitals and Intermolecular forces
4. Analysis of major chemical reactions that are used in the synthesis of molecules. 5. Understand the chemistry of various fuels and their combustion. Course Outcome
1. Describe and understand the operation of electrochemical systems for the production of electric energy, i.e. batteries.
2. Explain the mode by which potable water is produced through the processes of screening, micro Straining, aeration, coagulation and flocculation, sedimentation, flotation, filtration and disinfection.
3. Recognize that molecular orbital theory is a method used by chemists to determine the energy of the electron in a molecule as well as its geometry.
4. Demonstrate an ability to design, implement, and evaluate the results of experimentation using standard scientific methodologies such as hypothesis formulation and testing.
5. Understand and analyze the combustion mechanisms of various fuels
- Teacher: Dr. Naresh Kumar Assistant Professor
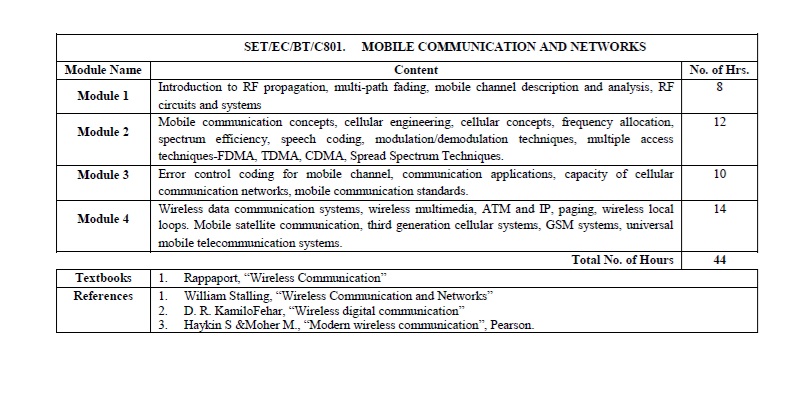
Course Objectives:
1. To understand the basic concepts of RF propagation, circuits and systems.
2. To understand the various modulation/demodulation techniques and multiple access techniques.
3. To study about mobile communication standards and applications.
Course Outcomes:
Student should be able to:
1. Understand the concepts of RF propagation circuits and systems.
2. Explain multiple access techniques-FDMA, TDMA, CDMA, etc.
3. Analyze wireless data communication systems, wireless multimedia, and GSM systems.
- To introduce the student to the basics of wave optics, lasers, and demonstrate their applications in technology, and make them aware of quantum physics phenomena. Further, a brief introduction of recent developments in materials science & engineering and its application within the class framework. Also Introduces the relationship between processing, structure, and physical properties of materials engineering. Finally, make them aware of Electromagnetic waves and the fundamentals of electromagnetism.
- Teacher: Dr. Om Prakash Assistant Professor
Semester VI
Credits- 06
Major Paper
Elective 1 [A]
Dimensions of Philosophy of Religion
1. Religious Consciousness
2. Religious Knowledge
3. Religious Experiences
4. Religious Tolerance
5. Religious Unity
6. Religious Diversity
BOOKS RECOMMENDED
1. Philosophy of Religion : J.H.Hick
Dharma Darshana : ( Hindi Translation of Philosophy of Religion)
Hindi Madhyam Karyanvaya Nideshalaya
2. Modern philosophy of Religion : A Thomson
3. Philosophy of Religion : Yakub Masih
4. Philosophy of Religion : C. Meister
5. Euthyphro : Plato (Translated by C. J. Emlyn-Jones)
6. Dharma Darshan Ke Mool Siddhant : V. P. Verma
BA III Year
Credit -06
Semester- V
Major Paper
Elective- 1 [A]
Philosophy of Religion
1. Definition and Nature of Religion, Relation between Philosophy and Religion
2. God, Attributes of God
3. Importance of Faith, Utility of Prayer
4. Arguments for the Immortality of the Soul
5. Arguments for the Existence of God
6. Arguments for the Rejection of God
BOOKS RECOMMENDED
1. Philosophy of Religion : J.H.Hick
Dharma Darshana : ( Hindi Translation of Philosophy of Religion)
Hindi Madhyam Karyanvaya Nideshalaya
2. Modern philosophy of Religion : A Thomson
3. Philosophy of Religion : Yakub Masih
4. Philosophy of Religion : C. Meister
5. Euthyphro : Plato (Translated by C. J. Emlyn-Jones)
6. Dharma Darshan Ke Mool Siddhant : V. P. Verma
Semester IV
Multidisciplinary- 2
Credit : 4
Vedic Value System- 2
1. Varṇa Vyavasthā
2. Āśrama Vyavasthā
3. Puruṣārtha
4. Ṛt, Ṛṇa,Yaj᷈an, Ṣoḍaśa Saṁskāra
BOOKS RECOMMEENDED
1. Vedanta Sar : Sadanand Yogi
2. Indian Philosophy : S. Radhakrishnan
3. Elements of Moral Philosophy : James Rachels
4. Moral Laws : H.J. Paton
5. The Indian Conception of Values : M. Hiriyana
6. Ethical Philosophies in India : I.C. Sharma
7. Transform Yourself : Kavita Bhatt
8. नीतिशास्त्र के मूल सिद्धान्त : वेद प्रकाश वर्मा
9. नीतिशास्त्र की समकालीन प्रवृतियाँ : सुरेन्द्र वर्मा
10. नीतिशास्त्र : जे. एन. सिन्हा
11. नीतिशास्त्र का सर्वेक्षण : संगम लाल पाण्डेय
12. पाश्चात्य नीतिदर्शन : मुकेश चन्द्र डिमरी
13. भारतीय नीतिशास्त्र : बी0एल0 आत्रेय
14. नैतिकता एवं सामाजिक विसंगतियाँ : मुकेश चन्द्र डिमरी
15. नीतिशास्त्र की रूपरेखा : अशोक कुमार वर्मा
16. योग परम्परा में प्रत्याहार: आध्यात्मिक, दार्शनिक एवं व्यावहारिक परिप्रेक्ष्य : कविता भट्ट
17. योग साधना : कविता भट्ट
18. गीता वदति : सती शंकर, कविता भट्ट
19. सांख्य एवं शंकर वेदांत का तुलनात्मक अध्ययन : ऋषिका वर्मा
20. योग के विविध आयाम ऋषिका वर्मा
Major Core Paper - 1
Credits-06
British Empiricists and German Idealist
1. John Locke: Refutation of Innate Ideas, Theory of Knowledge
2. George Berkeley: Rejection of Distinction Between Primary and Secondary Qualities, Idealism
3. David Hume: Rejection of Universal Causal Relation, Skepticism
4. Emanuel Kant: Space and Time, Categories of Understanding
5. Emanuel Kant: Possibility of Synthetic A-Priori Judgment, Reconciliation of Rationalism and Empiricism
6. G. W. F. Hegel: Dialectical Method, Absolute Idealism
BOOKS RECOMMENDED
1. A Critical History of Western Philosophy : D.J.O. Corner
2. History of Modern Philosophy : W.K. Wright
3. Hundred Years of Philosophy : John Passmore
4. A Student's History of Philosophy : Rogers Scruton
5. A History of Philosophy : From Descartes
to Wittgenstein : Rogers Scruton
6. Hume and Kant on Knowledge : Indoo Pandey
7. An Introduction to Philosophy : J.N. Sinha
8. An Introduction to Philosophy : Bali
9. पाश्चात्य दर्शन : चन्द्रधर शर्मा
10. आधुनिक दर्शन की भूमिका : संगम लाल पाण्डेय
11. पाश्चात्य दर्शन : दयाकृष्ण
12. पाश्चात्य दर्शन : याकूब मसीह
13. पाश्चात्य दर्शन : बी. एन. सिंह
14. दर्शन की धाराएँ : अर्जुन मिश्र
15. पाश्चात्य दर्शन : जयदेव सिंह
16. पाश्चात्य दर्शन : आर. एन. शर्मा
Semester III Credits-04
Additional Multidisciplinary- 1
Vedic Value System- 1
1. Vedic System of Values
2. The Pañćakośa theory of Vyaktitva
3. Ethical Concepts: Iṣṭa-Aniṣṭa, Śubha-Aśubha, Śreyas-Preyas
4. Concept of Dharma and Svadharma
BOOKS RECOMMENDED
1. The Philosophy of Nyaya Vaisesika : D.N. Shastri
2. Indian Realism : D.N. Shastri
3. Basic Ways of Knowing : G. Bhatta
4. Tarka Samgraha : Annam Bhatta
5. Vedanta Sar : Sadanand Yogi
6. Indian Philosophy : S. Radhakrishan
7. Nyaya Manjari, Jayant Bhatt : Translated by
S.R.Bhatt & Shashi Prabha
8. Transform Yourself : Kavita Bhatt
9. गीता वदति : सती शंकर, कविता भट्ट ‘शैलपुत्री
10. योग साधना : कविता भट्ट
1. सांख्य एवं शंकर वेदांत का तुलनात्मक अध्ययन : ऋषिका वर्मा
12. योग के विविध आयाम : ऋषिका वर्मा
Semester III Credits-04
Additional Multidisciplinary- 1
Vedic Value System- 1
1. Vedic System of Values
2. The Pañćakośa theory of Vyaktitva
3. Ethical Concepts: Iṣṭa-Aniṣṭa, Śubha-Aśubha, Śreyas-Preyas
4. Concept of Dharma and Svadharma
BOOKS RECOMMENDED
1. The Philosophy of Nyaya Vaisesika : D.N. Shastri
2. Indian Realism : D.N. Shastri
3. Basic Ways of Knowing : G. Bhatta
4. Tarka Samgraha : Annam Bhatta
5. Vedanta Sar : Sadanand Yogi
6. Indian Philosophy : S. Radhakrishan
7. Nyaya Manjari, Jayant Bhatt : Translated by
S.R.Bhatt & Shashi Prabha
8. Transform Yourself : Kavita Bhatt
9. गीता वदति : सती शंकर, कविता भट्ट ‘शैलपुत्री
10. योग साधना : कविता भट्ट
1. सांख्य एवं शंकर वेदांत का तुलनात्मक अध्ययन : ऋषिका वर्मा
12. योग के विविध आयाम : ऋषिका वर्मा
B.A II Year
Semester- III
Major Core Paper -I Credits- 06
History of Greek and Rationalist Philosophy
1. Socrates: Dialectical Reasoning, Knowledge and Virtue
2. Plato: Opinion and Knowledge, Theory of Ideas
3. Aristotle: Four Types of Causes; Types of Categories
4. Descartes: Method of Doubt, Problem of Dualism
5. Spinoza: Substance, Attributes and Modes, Parallelism
6. Leibnitz: Theory of Monads, Pre-established Harmony
BOOKS RECOMMENDED
1. A Critical History of Greek Philosophy W.T. Stace
2. A Critical History of Western Philosophy D.J.O. Corner
3. History of Modern Philosophy W.K. Wright
4. Hundred Years of Philosophy John Passmore
5. A Student's History of Philosophy Rogers Scruton
6. A History of Philosophy : From Descartes to Wittgenstein Rogers Scruton
7. Hume and Kant on Knowledge Indoo Pandey
8. An Introduction to Philosophy J.N. Sinha
9. An Introduction to Philosophy Bali
10. पाश्चात्य दर्शन चन्द्रधर शर्मा
11. आधुनिक दर्शन की भूमिका संगम लाल पाण्डेय
12.पाश्चात्य दर्शन दयाकृष्ण
13.पाश्चात्य दर्शन याकूब मसीह
14.पाश्चात्य दर्शन बी.एन.सिंह
15. दर्शन की धाराएँ अर्जुन मिश्र
Semester -II Credits: 04
Additional Multidisciplinary Course-2
Vedic Value System- 2
1. Varṇa Vyavasthā
2. Āśrama Vyavasthā
3. Puruṣārtha
4. Ṛt, Ṛṇa, Yaj᷈an, Ṣoḍaśa Saṁskāra
BOOKS RECOMMEENDED
1. Vedanta Sar : Sadanand Yogi
2. Indian Philosophy : S. Radhakrishnan
3. Elements of Moral Philosophy : James Rachels
4. Moral Laws : H.J. Paton
5. The Indian Conception of Values : M. Hiriyana
6. Ethical Philosophies in India : I.C. Sharma
Course Code: SOS-PHIL –P.G.-E-404 (A) Credit-3
Western Metaphysics
Unit I |
Theories of Metaphysical Entities |
A. Substance |
|
B. Attributes |
|
C. Modes
|
|
Unit II |
Theories of Mind-Body Relation |
|
A. Descartes |
|
B. Spinoza |
|
C. Leibnitz
|
Unit III |
Theories of Space and Time |
|
A. Aristotle |
|
B. Leibnitz |
|
C. Kant
|
Unit IV |
Theories of Causation |
|
A. Aristotle |
|
B. Hume |
|
C. Kant
|
BOOKS RECOMMENDED
1. Theory of Knowledge : R. Chislom
2. Knowledge : K. Lehrer.
3. An Introduction to Philosophical Analysis : J. Hospars.
4. Theory of Knowledge : A.D. Woozley.
5. Theory of Knowledge : D.W. Hamleyn.
6. History of Western Philosophy : Falken Berag
7. The Problems of Philosophy : Bertrand Russell.
8. The Concept of Mind : Gilbert Ryle.
9. Hume and Kant on Knowledge : Indoo Pandey.
10. An Introduction to Philosophy : J.N. Sinha
11. An Introduction to Philosophy : D.R. Bali
12. Western Philosophy : C.D. Sharma
Course Code: SOS-PHIL –P.G.-C-401
Credit-3
Contemporary Western Thinkers
Unit I |
Wittgenstein |
A. Functions of Philosophy |
|
B. Language – Game |
|
C. Logical Atomism
|
|
Unit II |
Edmund Husserl |
|
A. Meaning and Nature of Phenomena |
|
B. Phenomenological Reduction |
|
C. Bracketing Method
|
Unit III |
William James |
|
A. Pragmatic Theory of Truth. |
|
B. Radical Empiricism |
|
C. Concept of Self
|
Unit IV |
A.J. Ayer |
|
A. Functions of Philosophy |
|
B. Elimination of Metaphysics |
|
C. Verification Theory of Truth
|
BOOKS RECOMMENDED
1. Philosophical Investigations : Wittgenstein.
2. Tractatus- Logico- Philosophicus : Wittgenstein.
3. Hundred years of Philosophy : John Passmore.
4. Contemporary Western Philosophy : B.K. Lal.
5. Recent Developments in
Analytical Philosophy : R.C Pradhan.
6. Word Philosophies : Ninian Smart
Paper IV (A) SOS-PHIL –P.G.-E-304(A) Credit-3
Western Epistemology
Unit I |
Nature of Knowledge |
A. Definition & Kinds |
|
B. Knowing How & Knowing That |
|
C. Knowledge by Acquaintance & Knowledge by Description
|
|
Unit II |
Skepticism and Justification of Knowledge Claims |
|
A. Truth |
|
B. Belief |
|
C. Philosophical Skepticism
|
Unit III |
Theories of Knowledge |
|
A. Rationalism |
|
B. Empiricism |
|
C. Kantian Theory
|
Unit IV |
Theories of Truth |
|
A. Correspondence |
|
B. Coherence |
|
C. Pragmatic
|
BOOKS RECOMMENDED
1. Theory of Knowledge : R. Chislom
2. Knowledge : K. Lehrer.
3. An Introduction to Philosophical Analysis : J. Hospars.
4. Theory of Knowledge : A.D. Woozley.
5. Theory of Knowledge : D.W. Hamleyn.
6. History of Western Philosophy : Falken Berag
7. The Problems of Philosophy : Bertrand Russell.
8. The Concept of Mind : Gilbert Ryle.
9. Hume and Kant on Knowledge : Indoo Pandey.
10. An Introduction to Philosophy : J.N. Sinha
11. An Introduction to Philosophy : D.R. Bali
12. Western Philosophy : C.D. Sharma
Course Code: SOS-PHIL –P.G.-C-301
Credit-3
Theories of Contemporary Western Philosophy
Unit I
|
Theories of Realism |
A. Locke |
|
B. Moore |
|
C. Russell
|
|
Unit II |
Theories of Dialectical Philosophy |
|
A. Hegel |
|
B. Karl Marx |
|
C. A Comparative Account
|
Unit III |
Theories of Idealism |
|
A. Bradley |
|
B. Green |
|
C. Bosanquet
|
Unit IV |
Theories of Existentialism |
|
A. Kierkegarrd |
|
B. Sartre |
|
C. Neitzsche
|
BOOKS RECOMMENDED
1. Philosophical Investigations : Wittgenstein.
2. Tractatus- Logico- Philosophicus : Wittgenstein.
3. Existentialism: J.P Sartre.
4. Hundred years of Philosophy : John Passmore.
5. Contemporary Western Philosophy : B.K. Lal.
6. Recent Developments in
Analytical Philosophy : R.C Pradhan.
7. World Philosophies : Ninian Smart
8. Contemporary Westren Philosophy : RN Sharma
Course Code: SOS-PHIL –P.G.-C-203 Credit-3
Problems of Modern Western Philosophy
Unit I |
Locke |
A. Refutation of Innate Ideas |
|
B. Primary and Secondary Qualities |
|
C. Knowledge and Its Grades
|
|
Unit II |
Berkeley |
|
A. Esse-est-percipii |
|
B. Rejectioin of the Destinction between Primary and Secondary Qualities. |
|
C. Idealism
|
Unit III |
Hume |
|
A. Skepticism |
|
B. Causation |
|
C. Refutation of Self and God
|
Unit IV |
Kant |
|
A. The Synthetic a-priori judgments |
|
B. Space and Time |
|
C. Phenomena and Noumena
|
BOOKS RECOMMENDED
1. A Critical History of Greek Philosophy : W.T. Stace
2- A Critical History of Western Philosophy : D.J.O. Corner
3. History of Modern Philosophy : W.K. Wright
4. Hundred Years of Philosophy : John Passmore
5. A Student's History of Philosophy : Rogers Scruton
6. A History of Philosophy : From Descartes
to Wittgenstein : Rogers Scruton
7. Hume and Kant on Knowledge : Indoo Pandey
8. An Introduction to Philosophy : J.N. Sinha
9. An Introduction to Philosophy : D.R. Bali
Course Code –P.G.-C-204
Credit-3
Philosophical Perspectives of Modern Indian Thinkers
Unit I |
Philosophical Perspectives of Radhakrishnan |
A. God |
|
B. Man and Nature |
|
C. Religion and Religious Experience
|
|
Unit II |
Philosophical Perspectives of M.K. Ghandhi |
|
A. Concept of Truth |
|
B. Non-Violence |
|
C. Peace and Universal Religion
|
Unit III |
Philosophical Perspectives of M.N. Roy |
|
A Materialism |
|
B. Critique of Religion |
|
C. Radical Humanism
|
Unit IV |
Philosophical Perspectives of Swami Dayanand Sarswati |
|
A. The Concept of Truth |
|
B Criticism of Caste System |
|
C. Philosophy of Education
|
BOOKS RECOMMENDED
1. Contemporary Indian Philosophy : B.K. Lal.
2. Gandhi &Social Philosophy : B.N. Ganguli.
3. The Philosophy of Gandhi-
A Study of his Basic Ideas : Glyn Richard.
4. Indian Philosophy : Dr. Radha Krishnan
5. A Critical Survey of Indian Philosophy : C.D. Sharma
6- A Cotemporary Metaphysics : H.M. Joshi
7. A History of Indian Philosophy (Vol. 1-5) : S.N. Das Gupta
Course Code: SOS-PHIL –P.G.-C-102 Credits: 3
Theories of Western Ethics
Unit I |
Definition, Nature& Scope of Ethics |
A. Definition of Ethics |
|
B. Nature of Ethics |
|
C. Scope of Ethics
|
|
Unit II |
Theories of Ethics |
|
A. Emotivism |
|
B. Utilitarianism |
|
C. Intuitionism
|
Unit III |
Kant’s Ethical Theory |
|
A. Categorical Imperatives |
|
B. Postulates of Morality |
|
C. Highest Good
|
Unit IV |
Nature of Values and Virtues |
|
A. Definition and Nature of Values |
|
B. Value-Neutrality and Culture specific value |
|
C. Moral and Intellectual Virtues |
BOOKS RECOMMENDED
1. Elements of Moral Philosophy : James Rachels
2. Five Types of Ethical Theory : C.D. Broad
3. Principia Ethica : G.E Moore
4. Moral Laws : H.J. Paton
5. Virtue Ethics : Rosalind Hursehouse
6. Language, Truth and Logic : A.J. Ayer
7. The Indian Conception of Values : M. Hiriyana
8. Ethical Philosophies in India : I.C. Sharma
9. NIchomachean Ethics : Aristotle
Course Code: SOS-PHIL –P.G.-C-103 Credits: 3
Problems of Vedic and Atheist Systems of Indian Philosophy
Unit I |
Veda & Upanishad |
A. Rta and Rna |
|
B. Atman and Brahman |
|
C. Moksa and Anand
|
|
Unit II |
Charvaka |
|
A. Pramana Mimamsa |
|
B. Materialism |
|
C. Refutation of Vedic Spiritualism
|
Unit III |
Jainism |
|
A. Syadvada |
|
B. Anekantvada |
|
C. The Nature of Reality
|
Unit IV |
Buddhism |
|
A. Pratityasamutpadavada |
|
B. Anatmavada |
|
C. Sunyavada
|
BOOKS RECOMMENDED
1. The Philosophy of Nyaya Vaisesika : D.N. Shastri
2. Indian Realism : D.N. Shastri
3. Basic Ways of Knowing : G. Bhatta
4. The Navya- Nyaya Logic : Vibha Gaur
5. Tarka Samgraha : Annam Bhatta
6. The Primer of Indian Logic : S. Kuppuswami Shastri
7. Vedanta Sar : Sadanand Yogi
8. Indian Philosophy : S. Radhakrishan
9. Nyaya Manjari, Jayant Bhatt :Translated by
S.R.Bhatt&Shashi Prabha Kumar
Course Title: Theories of Western Ethics
Course Code: SOS-PHIL –P.G.-C-102 Credit: 3
Theories of Western Ethics
Unit I |
Definition, Nature& Scope of Ethics |
A. Definition of Ethics |
|
B. Nature of Ethics |
|
C. Scope of Ethics
|
|
Unit II |
Theories of Ethics |
|
A. Emotivism |
|
B. Utilitarianism |
|
C. Intuitionism
|
Unit III |
Kant’s Ethical Theory |
|
A. Categorical Imperatives |
|
B. Postulates of Morality |
|
C. Highest Good
|
Unit IV |
Nature of Values and Virtues |
|
A. Definition and Nature of Values |
|
B. Value-Neutrality and Culture specific value |
|
C. Moral and Intellectual Virtues |
BOOKS RECOMMENDED
1. Elements of Moral Philosophy : James Rachels
2. Five Types of Ethical Theory : C.D. Broad
3. Principia Ethica : G.E Moore
4. Moral Laws : H.J. Paton
5. Virtue Ethics : Rosalind Hursehouse
6. Language, Truth and Logic : A.J. Ayer
7. The Indian Conception of Values : M. Hiriyana
8. Ethical Philosophies in India : I.C. Sharma
9. NIchomachean Ethics : Aristotle
Teacher: Dr. Rishika Verma, Assistant Professor
Course title Vedic Value System- 02
Additional Multidisciplinary Course
Credits: 04
Maximum Marks: 100
(a) End-semester Examination: 70 Marks
(b) Sessional Assessment: 30
Duration of End- semester Examinationa: 2 Hour
1. Varṇa Vyavasthā
2. Āśrama Vyavasthā
3. Puruṣārtha
4. Ṛt, Ṛṇa, Yaj᷈an, Ṣoḍaśa Saṁskāra
BOOKS RECOMMEENDED
1. Vedanta Sar Sadanand Yogi
2. Indian Philosophy S. Radhakrishnan
3. Elements of Moral Philosophy James Rachels
4. Moral Laws H.J. Paton
5. The Indian Conception of Values M. Hiriyana
6. Ethical Philosophies in India I.C. Sharma
7. Transform Yourself : Kavita Bhatt
8. नीतिशास्त्र के मूल सिद्धान्त वेद प्रकाश वर्मा
9. नीतिशास्त्र की समकालीन प्रवृतियाँ सुरेन्द्र वर्मा
10. नीतिशास्त्र जे. एन. सिन्हा
11. नीतिशास्त्र का सर्वेक्षण संगम लाल पाण्डेय
12. पाश्चात्य नीतिदर्शन मुकेश चन्द्र डिमरी
13. भारतीय नीतिशास्त्र बी0एल0 आत्रेय
14. नैतिकता एवं सामाजिक विसंगतियाँ मुकेश चन्द्र डिमरी
15. नीतिशास्त्र की रूपरेखा अशोक कुमार वर्मा
16. योग परम्परा में प्रत्याहार: आध्यात्मिक, दार्शनिक एवं व्यावहारिक परिप्रेक्ष्य : कविता भट्ट
17. योग साधना : कविता भट्ट
18. गीता वदति : सती शंकर, कविता भट्ट
19. सांख्य एवं शंकर वेदांत का तुलनात्मक अध्ययन : ऋषिका वर्मा
20. योग के विविध आयाम ऋषिका वर्मा
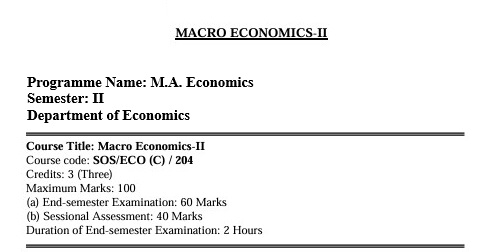
Course Title: Macro Economics-II
Course code: SOS/ECO (C) / 204
Credits: 3 (Three)
Maximum Marks: 100
(a) End-semester Examination: 60 Marks
(b) Sessional Assessment: 40 Marks
Duration of End-semester Examination: 2 Hours
SYLLABUS
Unit-1: Classical and Keynesian Macro Economics; Post Keynesian development in Macro
Economics: Monetarism; Supply-side Economics; New Classical Economics: The new classical
macroeconomic approach; Policy implications of new classical approach – Rational expectations
theory, Role of expectations in macroeconomic analysis.
Unit-2: Theory of Inflation: Classical, Keynesian and Monetarist approaches to inflation;
Structuralist theory of inflation; Philips curve analysis – Short run and long run Philips curve; the
nature rate of unemployment hypothesis; Tobin’s modified Philips curve and policies to control
inflation.
Unit-3: IS-LM model: The interaction of Real and Monetary sectors of the economy – Keynesian
version of the IS-LM model – Neo-classical version of the IS-LM model; Fiscal and Monetary
Policy analysis in an IS-LM model; Short run and long run Aggregate Supply curve analysis.
Unit-4: Business cycles: Meaning, phases and features; Theories of business cycles: Hawtrey
theory, Over-investment theory, Keynes theory, Samuelson model and Hick’s theory, Control of
business cycles, relative effectiveness of Monetary and fiscal policies Fiscal policy and crowding
out.
Recommended Reading List
- Ackley,G. (1978), Macroeconomics : Theory and Policy, Macmillan, New York
- Blackhouse, R. and A. Salansi (Eds.) (2000), Macroeconomics and the Real World (2 Vols.), Oxford University Press, London
- Branson, W.A. (1989), Macroeconomic Theory and Policy, (3rd Edition), Harper and Row, New York
- Dornbusch, R. and F. Stanley (1997), Macroeconomics, McGraw Hill, Inc., New York.
- Hall, R.E. and J.B. Taylor (1986), Macroeconomics, W.W. Norton, New York
- Heijdra, B.J. and V.P. Fredericck (2001), Foundations of Modern Macroeconomics, Oxford University Press, New Delhi
- Jha, R. (1991), Contemporary Macroeconomic Theory and Policy, Wiley Eastern Ltd., New Delhi
- Romer, D.L. (1996), Advanced Macroeconomics, McGraw Hill Company Ltd., New York Scarfe,
- B.L. (1977), Cycles, Growth and Inflation, McGraw Hill, New York
- Shapiro, E. (1996), Macroeconomic Analysis, Galgotia Publications, New Delhi
- Surrey, M.J.C. (Ed.) (1976), Macroeconomic Themes, Oxford University Press, Oxford
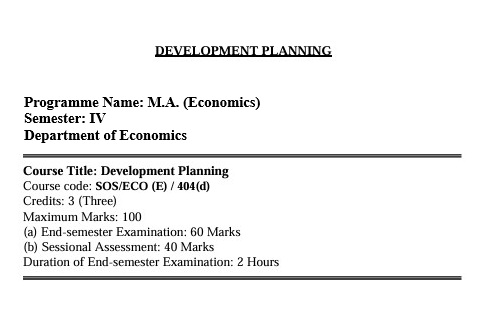
Course Title: Development Planning
Course code: SOS/ECO (E) / 404(d)
Credits: 3 (Three)
Maximum Marks: 100
(a) End-semester Examination: 60 Marks
(b) Sessional Assessment: 40 Marks
Duration of End-semester Examination: 2 Hours
SYLLABUS
Unit-1: Economic Planning: Economic Planning: Meaning, Need, objectives, limitations; Process
of Plan formation, requisites for successful planning; Types of planning. Planning under capitalism
and socialism; Planning in a mixed economy.
Unit-2: Planning and Market mechanism: Difference between planned and market economies;
Role and nature of prices in planned economies, nature of planned prices State intervention versus
liberalization and privatization; Development planning models; Capital-Output Ratio; Choice of
techniques.
Unit-3: Project planning: Formulation and implementation of project, problems in project
planning; Project evaluation: Project profitability analysis; Cost- benefit analysis; Shadow prices,
optimization in planning-Linear programming, manpower planning
Unit-4: Models in Indian Plan: From first to Ninth five year plan; factors leading to the adoption of
different models in different five year plans; Instruments of Indian Planning: Direct controls,
Indirect controls, redefining the role of the state.
Recommended Reading List
Unit-I:
Disciplinary lineage of IR, International Politics: Meaning, Nature, Scope and Development
Unit-II:
Realism and Neo-Realism
Liberalism and Neo-Liberalism
Unit- III:
English School
Constructivism
Unit- IV:
Marxist Theory
Feminism, Non-western IRT
- Teacher: Dr. Naresh Kumar Assistant Professor
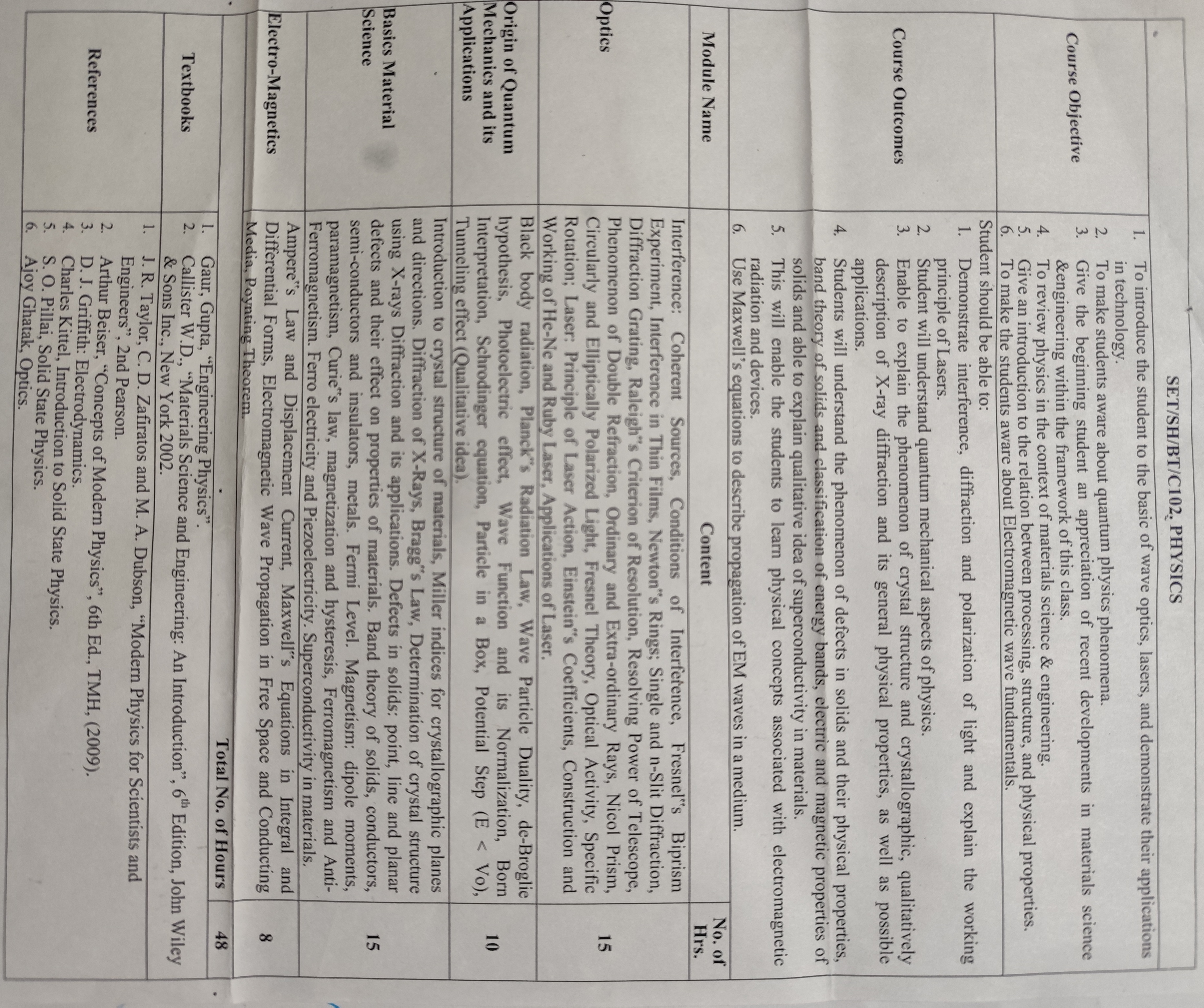
Course Objectives: |
|
Course Outcomes: |
|
- Teacher: Dr. Om Prakash Assistant Professor
Course Code: SOS-PHIL –P.G.-C-103 Credits: 3
Problems of Vedic and Atheist Systems of Indian Philosophy
Unit I |
Veda & Upanishad |
A. Rta and Rna |
|
B. Atman and Brahman |
|
C. Moksa and Anand
|
|
Unit II |
Charvaka |
|
A. Pramana Mimamsa |
|
B. Materialism |
|
C. Refutation of Vedic Spiritualism
|
Unit III |
Jainism |
|
A. Syadvada |
|
B. Anekantvada |
|
C. The Nature of Reality
|
Unit IV |
Buddhism |
|
A. Pratityasamutpadavada |
|
B. Anatmavada |
|
C. Sunyavada
|
BOOKS RECOMMENDED
1. The Philosophy of Nyaya Vaisesika : D.N. Shastri
2. Indian Realism : D.N. Shastri
3. Basic Ways of Knowing : G. Bhatta
4. The Navya- Nyaya Logic : Vibha Gaur
5. Tarka Samgraha : Annam Bhatta
6. The Primer of Indian Logic : S. Kuppuswami Shastri
7. Vedanta Sar : Sadanand Yogi
8. Indian Philosophy : S. Radhakrishan
9. Nyaya Manjari, Jayant Bhatt :Translated by
S.R.Bhatt&Shashi Prabha Kumar